C# - The right way to use iDisposable interface
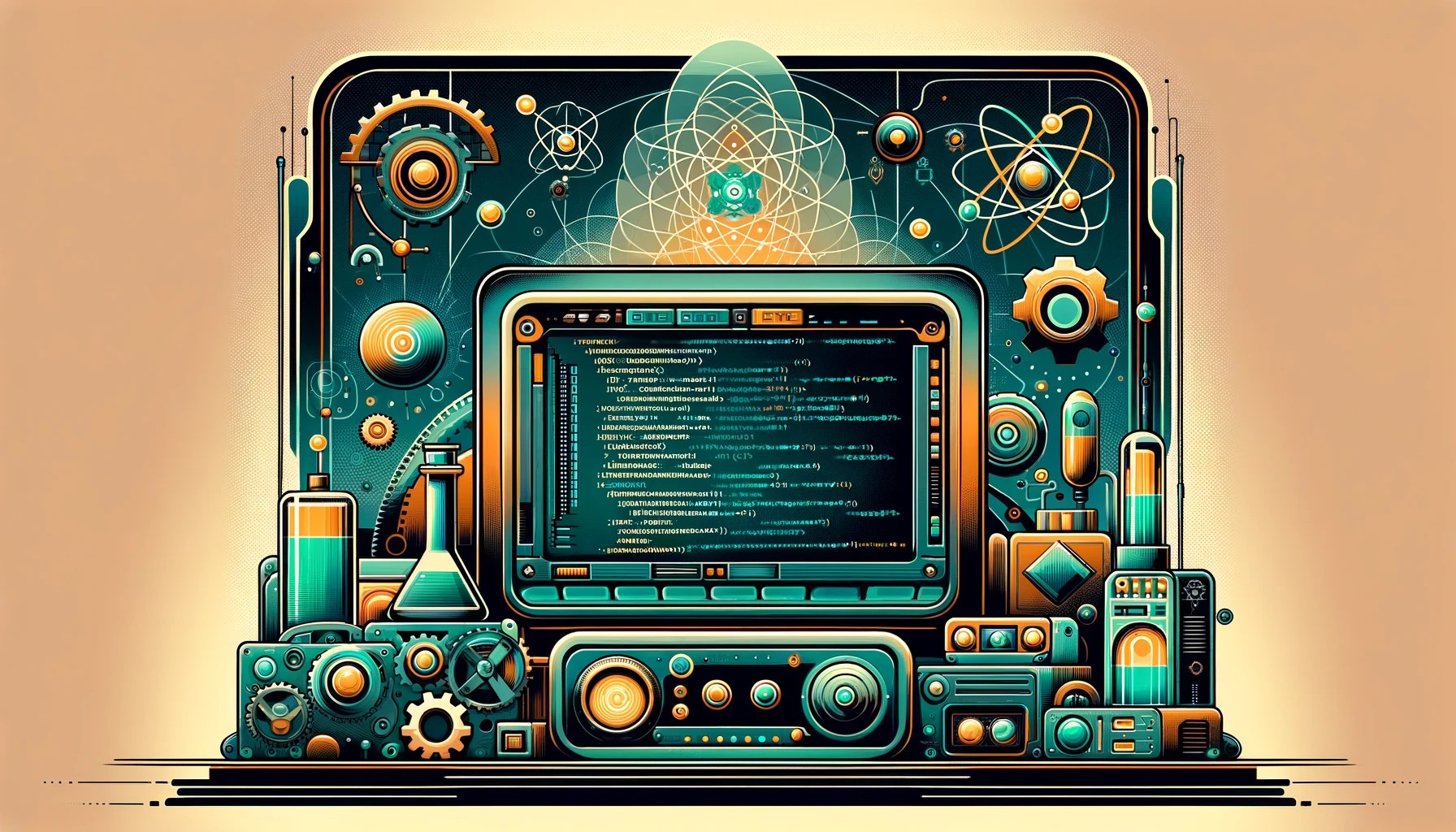
The IDisposable interface is one of the fundamental components of the .NET framework and represents an idiomatic pattern for managing unmanaged resources in C#. When an object implements this interface, it signals to the runtime that it owns unmanaged resources, such as file handles, network sockets, or other unmanaged objects, that need to be explicitly released.
The Dispose method defined in the IDisposable interface serves as a mechanism for releasing these unmanaged resources. When called, the Dispose method should release all unmanaged resources held by the object and restore it to a manageable state so that the object can be properly collected by the garbage collector.
Here's a simple example of a class that implements IDisposable:
using System; using System.IO; public class FileReader : IDisposable { private bool _disposed = false; private FileStream _fileStream; public FileReader(string path) { _fileStream = new FileStream(path, FileMode.Open); } public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } protected virtual void Dispose(bool disposing) { if (!_disposed) { if (disposing) { // Release managed resources _fileStream?.Dispose(); } // Release unmanaged resources // No unmanaged resources to release in this example _disposed = true; } } public string ReadFileContents() { if (_disposed) { throw new ObjectDisposedException(nameof(FileReader), "Cannot access a disposed object."); } using (var reader = new StreamReader(_fileStream)) { return reader.ReadToEnd(); } } ~FileReader() { Dispose(false); } }
This code defines a class called FileReader, which implements the IDisposable interface. The class is responsible for reading the contents of a file. Upon instantiation, it opens a file specified by the provided path using a FileStream.
The Dispose method is implemented to release both managed and unmanaged resources associated with the FileReader instance. Managed resources, in this case, refer to the FileStream object, which is disposed of to release any system resources it holds. Unmanaged resources, if any, would typically be released in this method as well, but in this example, there are none.
The ReadFileContents method reads the contents of the file using a StreamReader and returns it as a string. It also checks if the object has been disposed of before attempting to access the file, throwing an ObjectDisposedException if it has.
Finally, there's a finalizer (~FileReader) to ensure that resources are released if the object is not explicitly disposed of, but it's primarily there as a backup mechanism and not relied upon due to potential performance implications.
In the following example, we see how to use our new disposable class within a using block:
using System; class Program { static void Main(string[] args) { // Specify the path of the file to be read string filePath = "example.txt"; // Create a new instance of FileReader, which implements IDisposable using (var fileReader = new FileReader(filePath)) { try { // Read the contents of the file string fileContents = fileReader.ReadFileContents(); // Display the contents to the console Console.WriteLine("File contents:"); Console.WriteLine(fileContents); } catch (Exception ex) { // Handle any exceptions that occur during file reading Console.WriteLine($"An error occurred while reading the file: {ex.Message}"); } } } }
The using statement ensures that the FileReader object is properly released at the end of the block, even in the case of exceptions. This pattern is very common and recommended for using IDisposable objects.
When implementing IDisposable, it's important to follow some best practices:
-
> Implement the full disposal pattern: Use the full disposal pattern with Dispose and Dispose(bool) as shown in the previous example. This ensures proper resource management both when Dispose is called and when the object is finalized.
-
> Avoid unmanaged code in the implementation: The implementation of Dispose should be managed and not allocate unmanaged resources to avoid potential memory leaks.
-
> Handle exceptions: Handle exceptions within Dispose and ensure that resources are always released properly, even in the case of errors.
-
> Use using blocks when possible: Leverage using blocks to ensure that IDisposable objects are properly released, even in the presence of exceptions.
-
> Avoid calling Dispose on already disposed objects: Ensure that Dispose is not called multiple times on the same object, as it may cause unexpected behavior or exceptions.
By following these best practices, you can properly implement and use IDisposable, ensuring efficient management of unmanaged resources and preventing potential memory leaks and other resource-related issues.