C# - How to use Late Binding
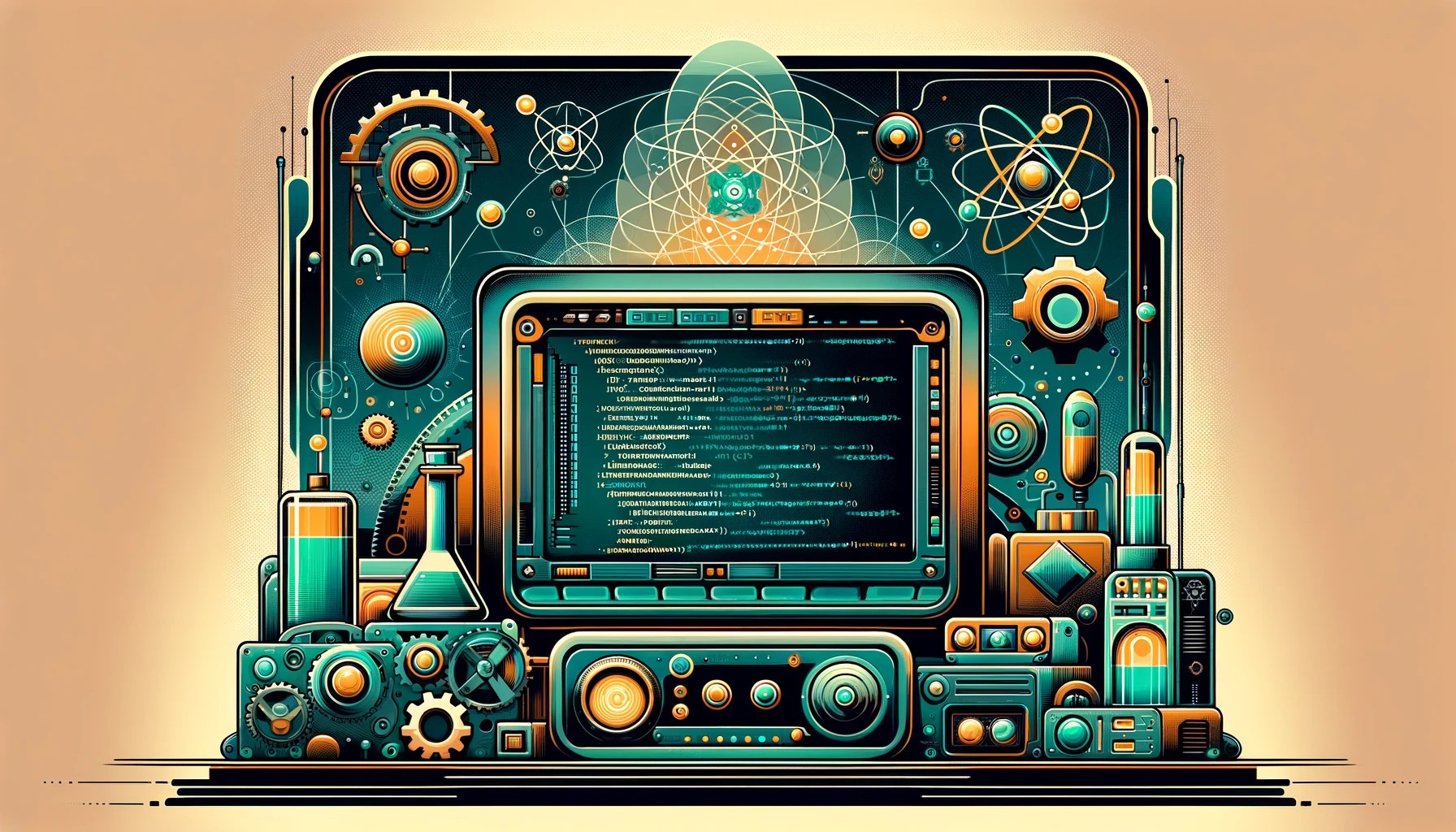
Late Binding in C# is a mechanism that allows resolving an object's members (methods, properties, events) at runtime instead of during compilation. It means that the binding between the object and its members occurs later than the normal Early Binding, which happens during the compilation phase.
Late Binding offers more flexibility compared to Early Binding, as it allows working with objects whose type is unknown at compile-time. However, this advantage comes with a performance cost, as Late Binding requires additional processing during runtime to resolve the object's members.
On the other hand, Early Binding associates objects with their members during the compilation phase. This means that the compiler can perform more in-depth checks and code optimizations, ensuring better performance compared to Late Binding. However, Early Binding requires the object's type to be known at compile-time, limiting code flexibility.
Late Binding in C# can be implemented using the Object type or the dynamic type introduced in C# 4.0. The Object type represents the base type of all objects in .NET and allows accessing an object's members dynamically through reflection. The dynamic type, on the other hand, offers a more readable syntax and allows writing code that appears to use Early Binding, but actually uses Late Binding under the hood.
In the following sections, we will explore practical examples of Late Binding in C# using both the Object type and the dynamic type, and discuss best practices to follow for proper use of this technique.
Dynamic Loading of an External Assembly
Let's assume we have an application that needs to dynamically load external libraries (assemblies) during runtime. These libraries contain classes with methods and properties that need to be used by the application, but the exact types are unknown at compile-time. In this scenario, Late Binding is the only good way to interact with the dynamically loaded classes.
// Load external assembly Assembly assembly = Assembly.LoadFrom("AssemblyName.dll"); // Get the target class type Type classType = assembly.GetType("NamespaceName.ClassName"); // Create an instance of the class object classInstance = Activator.CreateInstance(classType); // Access a class method using Late Binding MethodInfo targetMethod = classType.GetMethod("TargetMethod"); object result = targetMethod.Invoke(classInstance, null); // Access a class property using Late Binding PropertyInfo targetProperty = classType.GetProperty("TargetProperty"); object propertyValue = targetProperty.GetValue(classInstance, null);
In this example, the application loads an external assembly using Assembly.LoadFrom. It then obtains the target class type through GetType and creates an instance of this class using Activator.CreateInstance. Since the exact types of the class and its members are unknown at compile-time, Late Binding is used to access a method and a property of the class.
Late Binding occurs through the use of MethodInfo and PropertyInfo, which allow retrieving information about the class's methods and properties at runtime. The Invoke method is used to call the target method, while GetValue allows retrieving the value of the target property.
This approach is particularly useful when working with dynamic assemblies or third-party libraries whose types and members are not known until runtime, enabling the application to interact with them in a flexible manner.
Interacting with JSON Objects
One common scenario where Late Binding proves useful is when working with structured data in JSON format. Since the JSON format doesn't have a strongly-typed representation in C#, Late Binding allows interacting with JSON data in a flexible and dynamic manner.
Let's assume we have an application that receives JSON data from an external API. This data can have different structures depending on the context, making it difficult to define static classes to represent them. By using the dynamic type, we can work with this data dynamically:
using Newtonsoft.Json; // JSON data received from the external API string jsonData = "{\"name\":\"Mario\",\"age\":35,\"address\":{\"street\":\"Via Roma\",\"city\":\"Milan\"}}"; // Deserialize JSON data into a dynamic object dynamic userData = JsonConvert.DeserializeObject<dynamic>(jsonData); // Access object properties using Late Binding string name = userData.name; int age = userData.age; dynamic userAddress = userData.address; string street = userAddress.street; string city = userAddress.city;
In this example, we use the Newtonsoft.Json library to deserialize the JSON data into a dynamic object. Thanks to Late Binding, we can access the properties of the userData object dynamically, as if they were strongly-typed properties.
Late Binding allows working with JSON data in a flexible manner, without having to define static classes to represent the data structure. This approach can significantly simplify the code when working with data that has varying or unknown structures at compile-time.
Of course, it's important to pay attention to runtime errors that can occur when using Late Binding, as no static checks are performed during compilation. In this example, if the JSON data doesn't contain the specified properties, runtime errors will occur.
Overall, Late Binding provides a powerful tool for handling dynamic data structures, particularly when working with JSON data or external libraries whose types are unknown until runtime.
Best Practices for Using Late Binding
While Late Binding offers advantages in terms of code flexibility and adaptability, it's important to consider some best practices for the proper and safe use of this technique:
-
Use Late Binding only when necessary: Late Binding comes with a performance cost compared to Early Binding, as it requires additional processing at runtime. Therefore, it's recommended to use Late Binding only when strictly necessary, such as when working with dynamic code or structured data whose type is unknown at compile-time.
-
Prefer Early Binding when possible: When the object types are known at compile-time, it's preferable to use Early Binding to ensure better performance and code safety. Early Binding allows the compiler to perform static checks and code optimizations, reducing the risk of runtime errors.
-
Use the dynamic type to improve readability: Although the Object type allows implementing Late Binding, using the dynamic type can significantly improve code readability. The dynamic type offers a syntax similar to Early Binding, making the code more intuitive and easier to understand.
-
Handle runtime errors: Since Late Binding doesn't perform static checks during compilation, it's important to properly handle runtime errors that may occur. For example, it's necessary to implement proper exception handling to prevent the application from unexpectedly crashing.
-
Document the use of Late Binding: When using Late Binding in a project, it's important to clearly document the sections of code that use it. This documentation will help other developers understand the reason for using Late Binding and the precautions to take.
-
Consider using reflection libraries: If you anticipate frequent use of Late Binding, it may be helpful to evaluate the use of third-party reflection libraries, such as System.Reflection.Metadata or System.Reflection.Emit. These libraries can simplify the use of reflection and improve performance in some cases.
By following these best practices, you can leverage the advantages of Late Binding in a safe and efficient manner, minimizing the risks associated with this technique and ensuring high-quality, maintainable code over time.