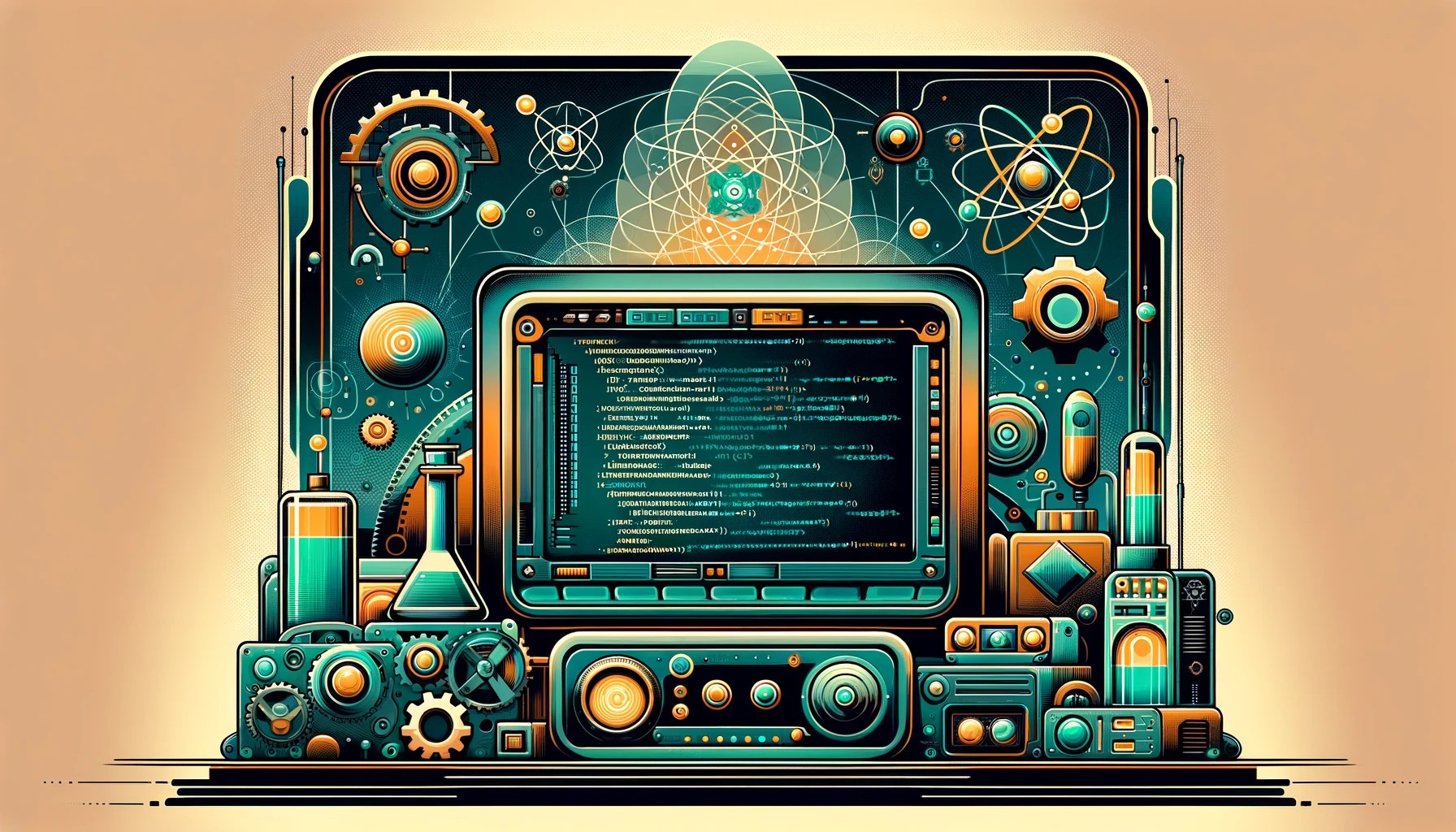
C# as a programming language offers three options for parameter passing in methods:'out', 'ref', and 'in'. These modifiers dictate how values are passed and handled within methods, impacting both the performance and readability of the code.
'ref' - Passing by Reference
The 'ref' modifier is used to pass an argument to a method by reference. This means any changes made to the parameter within the method are reflected in the original argument passed.
public void ModifyWithRef(ref int number) { number += 10; }
In this example, if number
initially is 5, after calling ModifyWithRef
, it will turn to 15.
'out' - Output Only
'out' is similar to 'ref' but is used when you want a method to return more than one value. The 'out' parameter must be modified in the method.
public void Calculate(int a, int b, out int sum, out int product) { sum = a + b; product = a * b; }
With 'out', there's no need to initialize the parameters before passing them to the method.
'in' - Read-Only
Introduced in C# 7.2, 'in' is a modifier indicating that a parameter is passed by reference but is intended to be used only for reading. This can enhance efficiency for large value types.
public void DisplayInParameter(in int number) { Console.WriteLine(number); }
With 'in', the method cannot modify the parameter's value.
Comparison and Practical Use
- Performance: 'in' is useful for improving performance when passing large structures, as it avoids the costly copying of values. 'ref' and 'out' are useful when there's a need to modify or return multiple values.
- Code Safety: 'in' ensures the method cannot modify the parameter, increasing the predictability of the code.
- Readability: The use of 'ref' and 'out' can make the code less readable, especially with many parameters. 'in' keeps the code cleaner when only needing to read the parameter.
Guidelines for Choosing Between 'ref', 'out', and 'in'
Choosing the right modifier in C# can significantly impact both the clarity of your code and its performance. Here are some guidelines on when to use 'ref', 'out', and 'in':
Using 'ref'
- Bidirectional Changes: Use 'ref' when both the calling and called method need to be able to read and modify the parameter's value.
- Performance with Large Data Types: If you're dealing with large data types (like structures) and need to modify them, 'ref' can be used to avoid unnecessary data copying.
- "Reference" Semantics: When you want to make it clear that the passed parameter is shared and subject to changes, 'ref' clearly conveys this intention.
Using 'out'
- Multiple Return Values: 'out' is ideal when your method needs to return more than one value. It's a common practice in C# to break a method into multiple output parts.
- Initialization Within the Method: If the parameter isn't initialized before the method call, 'out' is a suitable choice, as it enforces initialization within the method.
Using 'in'
- Passing Large Value Types for Reading: When you have large data structures that need to be only read, 'in' is the best choice. It reduces the performance overhead without the risk of accidental modifications.
- Clarity and Code Safety: If you want to ensure that the method doesn’t modify the parameter, 'in' provides this guarantee, improving the safety and predictability of your code.
Shortly, 'ref' is for read-write scenarios, 'out' is for returning additional values from a method, and 'in' is for passing large data for read-only purposes. The choice depends on the need to modify data, performance considerations, and semantic clarity of the code.