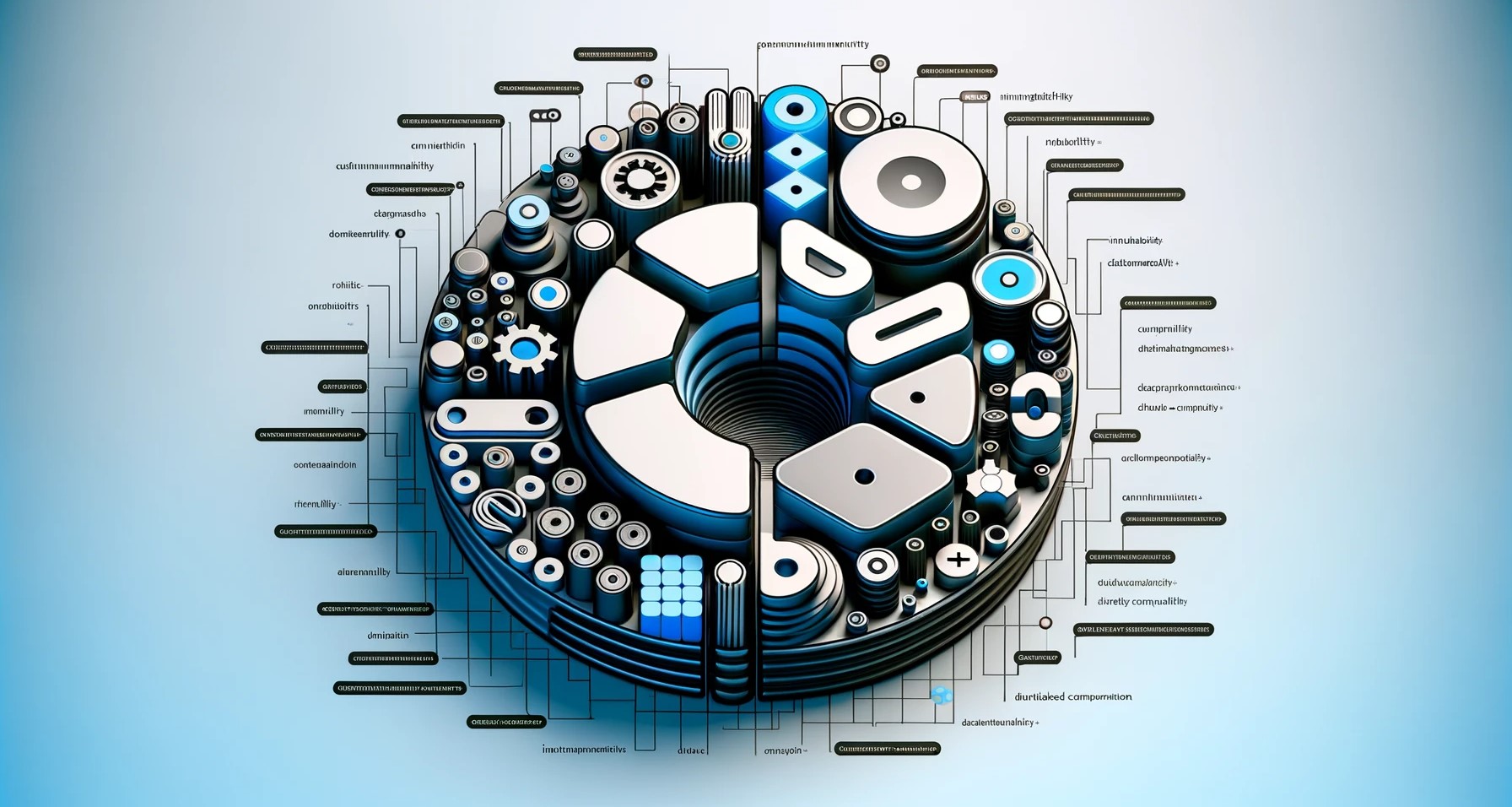
In C#, there are two similar data types that often cause confusion: records and structs. Both have their own advantages and disadvantages. Understanding exactly how they function is crucial for determining when and why to use one over the other. This article aims to clarify the differences between records and structs, providing practical guidelines to help developers make the right choice.
What are records in C#??
Introduced in C# 9.0, records are a reference type primarily intended to be immutable and to represent a set of data with semantic value. They are particularly useful for modeling data conceptually similar to values, where identity is not as important as the content.
Records key features:
- Immutability: The values of records cannot be changed after creation.
- Value-based Comparison: Two records are considered equal if all their fields have equal values.
- Simplified Syntax for Equality and Destructuring.
This is a record:
public record Person(string Name, int Age);
What are structs in C#?
In C#, structs are value types used to encapsulate data and behaviors related to small data structures. They are more suited for representing lightweight concepts with a small amount of data.
Structs key feature:
- Allocazione sullo stack: Le struct vengono generalmente allocate sullo stack, il che può portare a prestazioni migliori in alcuni scenari.
- Immutabilità non obbligatoria: A differenza dei record, le struct possono essere immutabili o mutabili.
- Confronto basato sull'identità: Per impostazione predefinita, il confronto tra due struct è basato sull'identità.
This is a struct:
public struct Point { public int X { get; } public int Y { get; } public Point(int x, int y) => (X, Y) = (x, y); }
When to Use Records vs Structs?
Use records when:
- You need to model immutable data.
- Object comparison is based on value rather than identity.
- You are working with more complex data structures that can benefit from features like inheritance.
Use struct when:
- You are managing small amounts of data.
- Performance is a key consideration, especially in scenarios with high allocation frequency.
- You prefer identity-based comparison or require complete control over the mutability of data.
The choice between records and structs in C# heavily depends on the specific context of the project. Records are ideal for representing immutable data with value-based comparison, while structs are the ideal choice in small data structures where performance and mutability are key considerations. Developers should carefully evaluate their specific needs and the characteristics of each type to make informed decisions that optimize the quality and efficiency of their code.